Hierarchical Data Grid Overview and Configuration
The Ignite UI for React Hierarchical Data Grid is used to display and manipulate hierarchical tabular data. Quickly bind your data with very little code or use a variety of events to customize different behaviors. This component provides a rich set of features like data selection, excel style filtering, sorting, paging, templating, column moving, column pinning, export to Excel and CSV, and more. The Hierarchical Grid builds upon the Flat Grid Component and extends its functionality by allowing the users to expand or collapse the rows of the parent grid, revealing corresponding child grids, when more detailed information is needed.
React Hierarchical Data Grid Example
In this React grid example you can see how users can visualize hierarchical sets of data and use cell templating to add other visual components.
Getting Started with Ignite UI for React Hierarchical Grid
Dependencies
To get started with the React hierarchical grid, first you need to install the igniteui-react
and igniteui-react-grids
packages.
npm install --save igniteui-react
npm install --save igniteui-react-grids
You also need to include the following import to use the grid:
import "igniteui-react-grids/grids/combined.js";
The corresponding styles should also be referenced. You can choose light or dark option for one of the themes and based on your project configuration to import it:
import 'igniteui-react-grids/grids/themes/light/bootstrap.css'
For more details on how to customize the appearance of the hierarchical grid, you may have a look at the styling section.
Using the React Hierarchical Data Grid
Data Binding
The IgrHierarchicalGrid derives from IgrGrid and shares most of its functionality. The main difference is that it allows multiple levels of hierarchy to be defined. They are configured through a separate tag within the definition of IgrHierarchicalGrid, called IgrRowIsland. The IgrRowIsland component defines the configuration for each child grid for the particular level. Multiple row islands per level are also supported. The Hierarchical Grid supports two ways of binding to data:
Using hierarchical data
If the application loads the whole hierarchical data as an array of objects referencing children arrays of objects, then the Hierarchical Grid can be configured to read it and bind to it automatically. Here is an example of a properly structured hierarchical data source:
export const singers = [{
"Artist": "Naomí Yepes",
"Photo": "assets/images/hgrid/naomi.png",
"Debut": "2011",
"Grammy Nominations": 6,
"Grammy Awards": 0,
"Tours": [{
"Tour": "Faithful Tour",
"Started on": "Sep-12",
"Location": "Worldwide",
"Headliner": "NO",
"Toured by": "Naomí Yepes"
}],
"Albums": [{
"Album": "Dream Driven",
"Launch Date": new Date("August 25, 2014"),
"Billboard Review": "81",
"US Billboard 200": "1",
"Artist": "Naomí Yepes",
"Songs": [{
"No.": "1",
"Title": "Intro",
"Released": "*",
"Genre": "*",
"Album": "Dream Driven"
}]
}]
}];
Each IgrRowIsland should specify the key of the property that holds the children data.
<IgrHierarchicalGrid data={singers} autoGenerate="true">
<IgrRowIsland childDataKey="Albums" autoGenerate="true">
<IgrRowIsland childDataKey="Songs" autoGenerate="true">
</IgrRowIsland>
</IgrRowIsland>
<IgrRowIsland childDataKey="Tours" autoGenerate="true">
</IgrRowIsland>
</IgrHierarchicalGrid>
[!NOTE] Note that instead of
data
the user configures only thechildDataKey
that the IgrHierarchicalGrid needs to read to set the data automatically.
Using Load-On-Demand
Most applications are designed to load as little data as possible initially, which results in faster load times. In such cases IgrHierarchicalGrid may be configured to allow user-created services to feed it with data on demand.
import { getData } from "./remoteService";
export default function Sample() {
const hierarchicalGrid = useRef<IgrHierarchicalGrid>(null);
function gridCreated(
rowIsland: IgrRowIsland,
event: IgrGridCreatedEventArgs,
_parentKey: string
) {
const context = event.detail;
const dataState = {
key: rowIsland.childDataKey,
parentID: context.parentID,
parentKey: _parentKey,
rootLevel: false,
};
context.grid.isLoading = true;
getData(dataState).then((data: any[]) => {
context.grid.isLoading = false;
context.grid.data = data;
context.grid.markForCheck();
});
}
useEffect(() => {
hierarchicalGrid.current.isLoading = true;
getData({ parentID: null, rootLevel: true, key: "Customers" }).then(
(data: any) => {
hierarchicalGrid.current.isLoading = false;
hierarchicalGrid.current.data = data;
hierarchicalGrid.current.markForCheck();
}
);
}, []);
return (
<IgrHierarchicalGrid
ref={hierarchicalGrid}
primaryKey="customerId"
autoGenerate="true"
height="600px"
width="100%"
>
<IgrRowIsland
childDataKey="Orders"
primaryKey="orderId"
autoGenerate="true"
gridCreated={(
rowIsland: IgrRowIsland,
e: IgrGridCreatedEventArgs
) => gridCreated(rowIsland, e, "Customers")}
>
<IgrRowIsland
childDataKey="Details"
primaryKey="productId"
autoGenerate="true"
gridCreated={(
rowIsland: IgrRowIsland,
e: IgrGridCreatedEventArgs
) => gridCreated(rowIsland, e, "Orders")}
></IgrRowIsland>
</IgrRowIsland>
</IgrHierarchicalGrid>
);
}
const URL = `https://data-northwind.indigo.design/`;
export function getData(dataState: any): any {
return fetch(buildUrl(dataState))
.then((result) => result.json());
}
function buildUrl(dataState: any) {
let qS = "";
if (dataState) {
if (dataState.rootLevel) {
qS += `${dataState.key}`;
} else {
qS += `${dataState.parentKey}/${dataState.parentID}/${dataState.key}`;
}
}
return `${URL}${qS}`;
}
Hide/Show row expand indicators
If you have a way to provide information whether a row has children prior to its expanding, you could use the HasChildrenKey
input property. This way you could provide a boolean property from the data objects which indicates whether an expansion indicator should be displayed.
<IgrHierarchicalGrid data={data} primaryKey="ID" hasChildrenKey="hasChildren">
</IgrHierarchicalGrid>
Note that setting the HasChildrenKey
property is not required. In case you don't provide it, expansion indicators will be displayed for each row.
Additionally if you wish to show/hide the header expand/collapse all indicator you can use the ShowExpandAll
property.
This UI is disabled by default for performance reasons and it is not recommended to enable it in grids with large data or grids with load on demand.
Features
The grid features could be enabled and configured through the IgrRowIsland markup - they get applied for every grid that is created for it. Changing options at runtime through the row island instance changes them for each of the grids it has spawned.
<IgrHierarchicalGrid data={localData} autoGenerate="false"
allowFiltering='true' height="600px" width="800px">
<IgrColumn field="ID" pinned="true" filterable="true"></IgrColumn>
<IgrColumnGroup header="Information">
<IgrColumn field="ChildLevels"></IgrColumn>
<IgrColumn field="ProductName" hasSummary="true"></IgrColumn>
</IgrColumnGroup>
<IgrRowIsland childDataKey="childData" autoGenerate="false" rowSelection="multiple">
<IgrColumn field="ID" hasSummary="true" dataType="number"></IgrColumn>
<IgrColumnGroup header="Information2">
<IgrColumn field="ChildLevels"></IgrColumn>
<IgrColumn field="ProductName"></IgrColumn>
</IgrColumnGroup>
<IgrPaginator perPage={5}></IgrPaginator>
<IgrRowIsland>
<IgrPaginator></IgrPaginator>
</IgrHierarchicalGrid>
The following grid features work on a per grid level, which means that each grid instance manages them independently of the rest of the grids:
- Sorting
- Filtering
- Paging
- Multi Column Headers
- Hiding
- Pinning
- Moving
- Summaries
- Search
The Selection and Navigation features work globally for the whole Hierarchical Grid
- Selection Selection does not allow selected cells to be present for two different child grids at once.
- Navigation When navigating up/down, if next/prev element is a child grid, navigation will continue in the related child grid, marking the related cell as selected and focused. If the child cell is outside the current visible view port it is scrolled into view so that selected cell is always visible.
Collapse All Button
The Hierarchical Grid allows the users to conveniently collapse all its currently expanded rows by pressing the "Collapse All" button at its top left corner. Additionally, every child grid which contains other grids and is a Hierarchical Grid itself, also has such a button - this way the user is able to collapse only a given grid in the hierarchy:
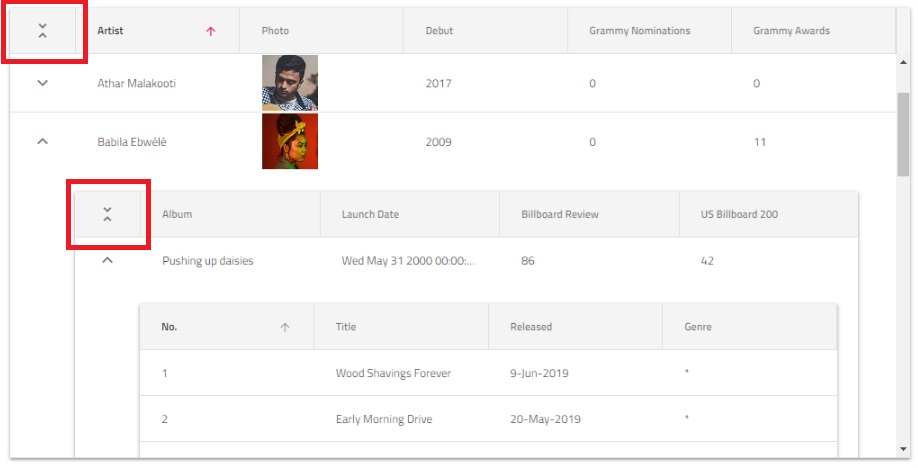
Styling
In addition to the predefined themes, the grid could be further customized by setting some of the available CSS properties. In case you would like to change the header background and text color, you need to set a class for the grid first:
<IgrHierarchicalGrid className="grid"></IgrHierarchicalGrid>
Then set the --header-background
and --header-text-color
CSS properties for that class:
.grid {
--header-background: #494949;
--header-text-color: #FFF;
}
Demo
Known Limitations
Limitation | Description |
---|---|
Group By | Group By feature is not supported by the hierarchical grid. |
API References
Our community is active and always welcoming to new ideas.